Quick Start Tutorial
This quick start walks you step-by-step through everything you need to get started. It'll take approximately 5 minutes. You will learn how to:
- Run Inngest on your machine for local testing
- Add Inngest to an existing Next.js project (other frameworks are also supported)
- Write your first function
- Trigger your function from the development UI
- Trigger your function from code
Afterwards you'll be ready to deploy your app to any platform, then build advanced functions using our guides! Let's get started.
1. Run Inngest locally
Before integrating Inngest we will start the open-source development server. Run the following command in your terminal:
shellnpx inngest-cli@latest dev
You should see the following output:
shell$ npx inngest-cli@latest dev12:38PM INF runner > starting event stream backend=inmemory12:38PM INF executor > starting queue backend=inmemory12:38PM INF devserver > autodiscovering locally hosted SDKs12:38PM INF executor > service starting12:38PM INF coreapi > service starting12:38PM INF devserver > service starting12:38PM INF executor > subscribing to function queue12:38PM INF runner > starting queue backend=inmemory12:38PM INF coreapi > starting server addr=0.0.0.0:830012:38PM INF api > starting server addr=0.0.0.0:828812:38PM INF runner > initializing scheduled messages len=012:38PM INF runner > service starting12:38PM INF runner > subscribing to events topic=eventsInngest dev server online at 0.0.0.0:8288, visible at the following URLs:- http://127.0.0.1:8288 (http://localhost:8288)
This runs Inngest locally. We'll use this to test our functions as we write them. With this running you can visit http://localhost:8288
to see the development UI:
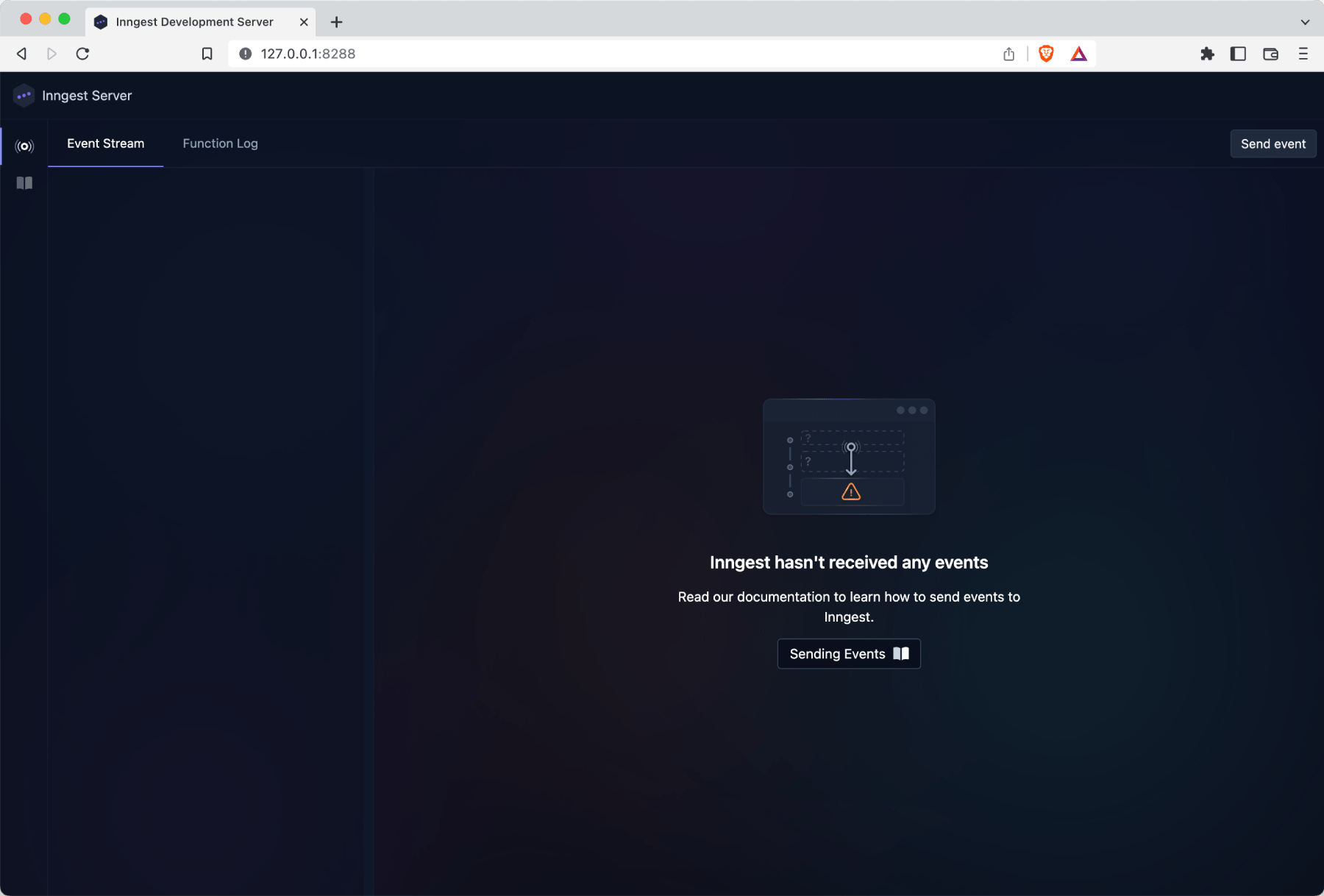
Let's get started with adding Inngest to a project!
2. Add Inngest to a Next.js project
Inngest runs your functions securely via an API endpoint at /api/inngest
. The first step is to add this API endpoint to your project.
Prerequisite: Create a new Next.js project
Let's start a new Next.js project by running create-next-app
in your terminal:
shellnpx create-next-app@latest --ts --eslint inngest-guide
You can answer the questions however you'd like. This creates a new inngest-guide
directory containing your project. Open this directory in your terminal and code editor, then we can start adding Inngest!
Install the Inngest SDK
Within your Next.js project root, add the Inngest JS SDK via NPM or Yarn:
shellnpm install inngest # or: yarn add inngest
Add the API handler
Create a new file at ./pages/api/inngest.ts
with the following code:
typescript// Within ./pages/api/inngest.tsimport { serve } from "inngest/next";// Create an API that hosts zero functions.export default serve("My app name", []);
Here, we're creating a new API endpoint with an application name and a list of available functions. You can import serve for other frameworks, though the code is the same — only the import changes.
This sets up the API endpoint for hosting and calling functions! We'll update the array passed in to serve
with functions as we write them.
3. Write your first function
Defining the function
Let's write your first reliable serverless function! Create a new file at ./inngest/demo.ts
- you will have to make a new folder. Within this file, add the following code:
typescriptimport { createStepFunction } from "inngest";export default createStepFunction("My first function", "test/demo", ({ event, tools }) => {tools.sleep("1 second");return { event, body: "hello!" }});
This creates a new function which will be called in the background any time Inngest receives an event with the name test/demo
. This introduces some new concepts:
- Functions are called by events (or on a schedule), in the background
- Functions can sleep
- Functions will retry automatically if they error.
Instead of calling your function directly as an API with a JSON body, you send a JSON event to Inngest. Inngest calls all functions listening to this event, and automatically passes the event payload JSON as an function argument.
Serving the function
Now that you've written your function let's make sure that you serve it from your Inngest API. Open up the API handler at ./pages/api/inngest.ts
and update the code with the following:
typescriptimport { serve } from "inngest/next";import demoFn from "../../inngest/demo"; // Import your functionexport default serve("My app name", [demoFn]); // Serve your function
Here, we're adding your new function to the array of functions that are served by the API. This enables Inngest to discover and call your functions in your app.
Now, let's run your function!
4. Triggering your function from the development UI
Ensure your Next.js app is up and running via npm run dev
inside project root, then head to http://localhost:8288
. You should see the following UI, which allows you to send events directly to Inngest:
To send the event, click on “Send event” in the top right corner then add the following event data:
json{"name": "test/demo","data": {"email": "test@example.com"}}
The event is sent to Inngest running locally via npx inngest-cli@latest dev
, which automatically runs your function in the background reliably!
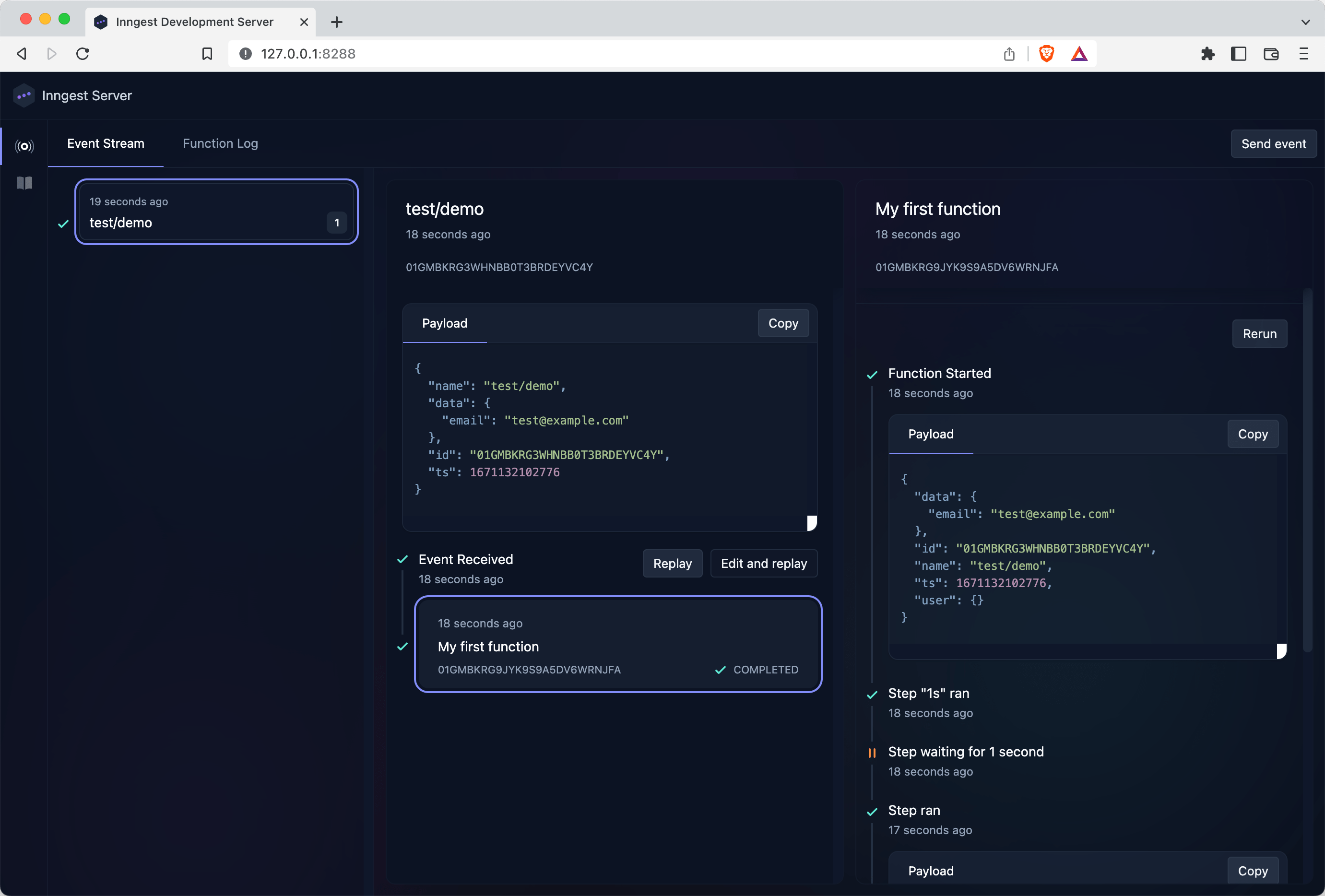
This highlights the power of event-driven development. Using events, you can:
- Run one or many functions automatically
- See the event payload, and use the event payload in your functions
- Store the event for some amount of time, to locally replay if there are errors in production
- Build complex sleeps and schedules without worrying about queues
There are many other benefits to using events. For now, let's show you how to trigger functions in your code by sending events from your own app.
5. Triggering your function from code
When you want to run functions reliably in your app, you'll need to send an event to Inngest. As you've seen, these events are forwarded to all functions that listen to this event.
To send an event from your code, you can use the Inngest
constructor and the send()
method. Let's send an event from the a “hello” Next.js API function that you can create in ./pages/api/hello.ts
:
typescriptimport { Inngest } from 'inngest';const inngest = new Inngest({ name: "My app name" });// Create a simple async Next.js API route handlerexport default async function handler(req, res) {// Send your event payload to Inngestawait inngest.send({name: "test/demo",data: {email: "test@example.com"}});res.status(200).json({ name: 'Hello Inngest!' });}
Every time this API route is requested, an event is sent to Inngest. To test it, drop this new API route's URL in your web browser: http://localhost:3000/api/hello
(change your port if you're app is running elsewhere). With the send
function you now can:
- Send one or more events within any API route
- Include any data you need in your function within the
data
object
In a real world app, you might send events from API routes that perform an action, like registering users (e.g. app/user.signup
) or creating something (e.g. app/report.created
).
That's it! You now have learned how to use Inngest by creating functions and sending events to trigger those functions. You can now explore how to deploy your app to your platform or learn how to use Inngest with other frameworks!
If you'd like to learn more about functions or sending events check out these related docs: