Using the CLI in 2 minutes
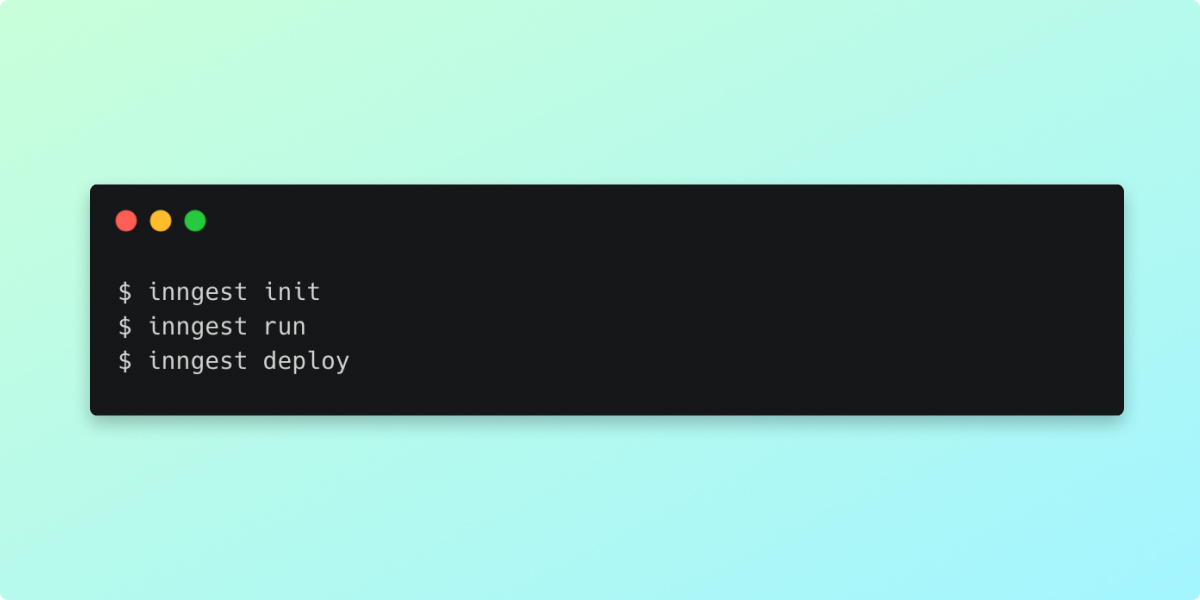
This guide explains how to initialize, develop, test, and then deploy your functions, with best practices and guidance, using the Inngest CLI.
The general flow of writing a serverless function using our CLI — which we’ll walk through in detail — is:
- Initialize a new function using
inngest init
- Add your logic to the function, depending on what the function needs to do
- Test the function by running it locally, using
inngest run
- Deploy the function to your host, using
inngest deploy
We’ll run through each of these steps beginning with installing the CLI. More advanced steps include setting up CI/CD; testing with historical, real-world data; monitoring your functions; and deploying to > 1 cloud provider.
Installing the CLI
To begin the process you’re going to need to install the CLI. The quickest and easiest method is by copying and pasting the following into your terminal:
curl -sfL https://cli.inngest.com/install.sh | sh \
&& sudo mv ./inngest /usr/local/bin/inngest
Alternatively, you can:
- Download the latest pre-built binary from our releases page here.
- Build the CLI from source, as long as you have Go installed.
Once installed, running inngest
from the CLI will print the following:
hi@inngest.com $ inngest
____ __
/ _/___ ____ ____ ____ _____/ /_
/ // __ \/ __ \/ __ '/ _ \/ ___/ __/
_/ // / / / / / / /_/ / __(__ ) /_
/___/_/ /_/_/ /_/\__, /\___/____/\__/
/____/
Usage:
inngest [command]
Available Commands:
completion Generate the autocompletion script for the specified shell
deploy Deploy a function to Inngest
help Help about any command
init Create a new serverless function
login Logs in to your Inngest account
run Run a serverless function locally
Flags:
-h, --help help for inngest
Use "inngest [command] --help" for more information about a command.
With the CLI installed, you can create your first function.
Creating your first function
Our CLI allows you to create a new serverless function using a single command. You can start developing in seconds by running inngest init
.
When you run inngest init
, you’ll be asked for the following questions:
- Your function name, for easily understanding your functions.
- A function trigger, which specifies the event that automatically triggers your function.
- A function language, using one of our pre-built templates.
We’ll guide you through the inngest init
process this guide’s function, and afterwards we’ll walk through what these options mean in detail.
Making your first function
Let’s go ahead and run inngest init
to create your function:
hi@inngest.com $ inngest init
⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼
⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼╭─────────────────────────────╮⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼
⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼│ │⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼
⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼│ 👋 Welcome to Inngest! │⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼
⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼│ │⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼
⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼╰─────────────────────────────╯⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼
⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼⎽⎼
Let's get you set up with a new serverless function.
Answer these questions to get started.
1. Function name:
→
For this guide, we’ll name the function “Post-payment flow”.
After you’ve entered your function name you’ll be prompted to select the event that triggers the function. In this case, type stripe/
and select the stripe/charge.succeeded
event. This function will be triggered any time this event is received in your Inngest account.
Finally, let’s select the “Typescript” language to use the typescript template. You should see the following:
gohi@inngest.com $ inngest initLet's get you set up with a new serverless function.Answer these questions to get started.1. Function name: Post-payment flow2. Event: stripe/charge.succeeded3. Language: typescript🎉 Done! Your project has been created in ./post-payment-flow## Next steps1. `cd` into your project at `./post-payment-flow`2. Run `npm install` to install the base dependences3. Add your logic within src/index.tsFor more information, read our documentation at https://www.inngest.com/docs
Your serverless function is ready to go in a new folder: ./post-payment-flow
.
Triggers
Serverless functions need to be invoked to run. In Inngest, functions are invoked by a trigger, which can be one of two things:
- An event, such as a JSON payload from your API, a webhook payload such as Stripe’s
charge.succeeded
event, someone signing in to your app, your own queuing system, an HTTP request, etc. - A cron schedule, allowing you to run functions repeatedly on a schedule
Specifying the trigger before you begin developing your function allows you to make sure you’re working with the right data, and it makes sure that your function doesn’t have bugs relating to data issues.
Languages
As well as specifying the trigger, you need to choose the language that you’ll use to write your serverless function.
The language you choose will be used to create the function’s bootstrapping logic, and we’ll create a Dockerfile for you to bundle and compile your function into a working image.
Writing and testing code
Once your project has been initialized, you can navigate to the directory containing your code to start writing your business logic. Each Inngest function has an inngest.json
or inngest.cue
file at the project’s root directory, which defines the function configuration:
tsx{// name represents the function's name"name": "Post-payment flow",// id is the function ID within Inngest"id": "sensible-ant-ac49c5",// triggers are a list of triggers that invoke the function"triggers": [{"event": "stripe/charge.succeeded","definition": {"format": "cue",// synced ensures that inngest is the source of truth."synced": true,"def": "file://./events/stripe-charge-succeeded.cue"}}]
In the Typescript template, your code begins execution within ./src/index.ts
. An empty project contains the following:
tsximport type { Args } from "./types";export async function run({ event }: Args) {// Your logic goes here.return event.name;}
Your serverless function is passed the event
as an argument, allowing your code to work with the incoming event’s data. You can install any packages you need to handle your business logic. For example, given you receive a new payment you can:
- Use node-fetch or axios to call other external APIs
- Send notifications via slack, discord, or email
- Create new purchase orders, shipments, or order fulfillment requests
- Store data in your own datastores eg. for recordkeeping, or ML
- Send the event to your own data warehouse
Locally testing your function
For now, we’ll run the entire project as-is. Running the command inngest run
in the project root will build your serverless function, then run it locally using mock data.
Let’s build and test the base project:
From here, you can make changes to ./src/index.ts
, then re-run inngest run
to compile and test your serverless function.
Deploying your function
When your function is ready to deploy, you can build and launch your function with a single command: inngest deploy
. The CLI will re-build your image (using buildx
), push a new version of the function to Inngest, and make this function version live.
You must be logged in to your Inngest account to fully deploy your function. First, run inngest login
to log in, and type your email address and password to log in.
With our upcoming Lambda compatibility, the CLI will deploy the function to your own Lambda account. Inngest itself will trigger your lambda function every time the event is received, or on the schedule you specify.
That’s it! With three commands — inngest init
, inngest run
, and inngest deploy
— you’ve built, locally tested, and deployed a serverless function, without writing any config or boilerplate code.